As a developer building interactive forms in React
applications are essential, but selecting the right form library can be overwhelming. Several powerful options exist, each with its own strengths and considerations. This post dives into a soft comparison of three popular choices: Formik
,
, and React Final Form
React Hook Form
. We’ll explore their key features, performance aspects, and use cases, equipping you to make an informed decision for your next React project in 2024.
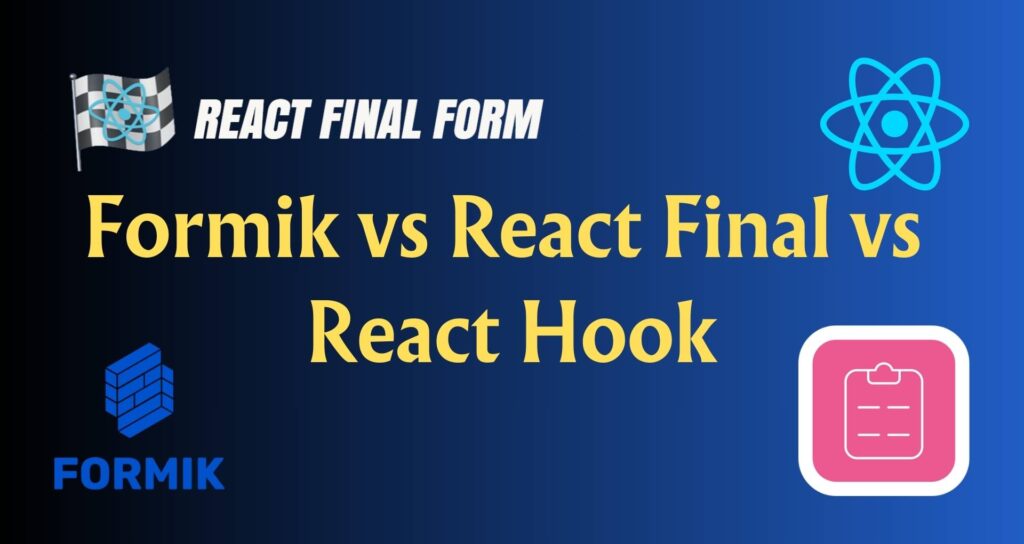
Formik
Build forms in React, without the tears.
- Official Web – https://formik.org/
- GitHub – https://github.com/jaredpalmer/formik
- NPM – https://www.npmjs.com/package/formik
Formik
is one of the most popular form libraries for React and React Native. With Formik’s
powerful features, you spend less time building powerful complex forms. Features are,
- It works well with both internal states and external state libraries.
- It provides well-integrated validations and supports external libraries like
Yup
. - It works well with controlled and uncontrolled form components.
- It is easy to customize the form and its behaviors.
Formik
has well-written documentation. It doesn’t have an active community as much React Hook Form
but it’s more active than React Final Form
(03/12/2024) in 2024. Formik
learning curve is not as easy as React Hook Form
but it doesn’t have a steep learning curve as React Final Form
.
But I found a few considerations,
Formik
has 690 open bugs on the GitHub when today (03/12/2024) – https://github.com/jaredpalmer/formik/issues.Formik
bundle size is larger thanReact Hook Form
.
Sample codes
// formik-form.js
import React from 'react';
import { ErrorMessage, Field, Form, Formik } from 'formik';
const FormikForm = () => {
const initialValues = {
firstName: '',
lastName: '',
email: '',
age: '',
};
// Validation
const validate = (values) => {
let errors = {};
if (!values.firstName) {
errors.firstName = 'First Name is Required';
}
if (!values.lastName) {
errors.lastName = 'Last Name is Required';
}
if (!values.age) {
errors.age = 'Age is Required';
} else if (!/^(0|[0-9][0-9]*)$/i.test(values.age)) {
errors.age = 'Age should be a number';
}
if (!values.email) {
errors.email = 'Email is Required';
} else if (!/^[A-Z0-9._%+-]+@[A-Z0-9.-]+\.[A-Z]{2,}$/i.test(values.email)) {
errors.email = 'Invalid email address';
}
return errors;
};
// handle submit
const onSubmit = (values, { setSubmitting }) => {
console.log(values);
setSubmitting(false);
};
return (
<>
<h3>Formik Form</h3>
<Formik
initialValues={initialValues}
validate={validate}
onSubmit={onSubmit}
>
{({ isSubmitting }) => (
<Form>
<label htmlFor="firstName">First Name</label>
<Field type="text" name="firstName" />
<ErrorMessage name="firstName" component="div" />
<label htmlFor="lastName">Last Name</label>
<Field type="text" name="lastName" />
<ErrorMessage name="lastName" component="div" />
<label htmlFor="age">Age</label>
<Field type="text" name="age" />
<ErrorMessage name="age" component="div" />
<label htmlFor="email">Email</label>
<Field type="email" name="email" />
<ErrorMessage name="email" component="div" />
<button type="submit" disabled={isSubmitting}>
Submit
</button>
</Form>
)}
</Formik>
</>
);
};
export default FormikForm;
Around a few years ago, Formik
was a better solution for form creation and I used it in my projects. But nowadays I can see Formik
is not as active as the React Hook Form
library. But I can say Formik
is still a powerful library for React forms.
Finally, my consideration is, Formik
is a solid powerful library that supports both react functional and class components. If you are looking for a library with well-written documentation this is an ideal solution.
React Final Form
High performance subscription-based form state management for React.
- Official Web – https://final-form.org/react
- GitHub – https://github.com/final-form/react-final-form
- NPM – https://www.npmjs.com/package/react-final-form
It’s a comprehensive React library specifically designed for building complex and flexible forms. It offers a rich set of features,
- It has built-in state management – Then we can handle form state without
Redux
. - It has integrated validation – This supports 3rd party validation libraries like
Yup
. - Subscription mechanism – This avoids unnecessary re-rendering ( https://codesandbox.io/p/sandbox/react-final-form-subscriptions-example-qigir?file=%2Findex.js ).
- It is flexible for connecting with
Redux
. - It supports custom validation.
As I think this React Final Form
is, Ideal for complex forms and complex validations. And It has a strong community and well-written documentation too.
But I found a few considerations,
- It has a steeper learning curve.
- It seems not updated recently in GitHub (12/03/2024).
Finally, My consideration is about the React Final Form
is a powerful library that supports building complex dynamic forms. If you require an advanced complex form this is an ideal solution. But please consider as I mentioned above it didn’t update recently.
Sample code
// react-final-form.js
import React from 'react';
import { Form, Field } from 'react-final-form';
const ReactFinalForm = () => {
const onSubmit = async (data) => {
console.log(data);
};
return (
<>
<h3>React Final Form</h3>
<Form
onSubmit={onSubmit}
initialValues={{ firstName: '' }}
validate={(values) => {
const errors = {};
if (!values.firstName) {
errors.firstName = 'Required';
}
if (!values.lastName) {
errors.lastName = 'Required';
}
if (!values.age) {
errors.age = 'Age is Required';
} else if (!/^(0|[0-9][0-9]*)$/i.test(values.age)) {
errors.age = 'Age should be a number';
}
if (!values.email) {
errors.email = 'Email is Required';
} else if (
!/^[A-Z0-9._%+-]+@[A-Z0-9.-]+\.[A-Z]{2,}$/i.test(values.email)
) {
errors.email = 'Invalid email address';
}
return errors;
}}
render={({ handleSubmit, form, submitting, pristine, values }) => (
<form onSubmit={handleSubmit}>
<Field name="firstName">
{({ input, meta }) => (
<div>
<label>First Name</label>
<input {...input} type="text" placeholder="First Name" />
{meta.error && meta.touched && <span>{meta.error}</span>}
</div>
)}
</Field>
<Field name="lastName">
{({ input, meta }) => (
<div>
<label>Last Name</label>
<input {...input} type="text" placeholder="Last Name" />
{meta.error && meta.touched && <span>{meta.error}</span>}
</div>
)}
</Field>
<Field name="age">
{({ input, meta }) => (
<div>
<label>Age</label>
<input {...input} type="text" placeholder="Age" />
{meta.error && meta.touched && <span>{meta.error}</span>}
</div>
)}
</Field>
<Field name="email">
{({ input, meta }) => (
<div>
<label>Email</label>
<input {...input} type="text" placeholder="Email" />
{meta.error && meta.touched && <span>{meta.error}</span>}
</div>
)}
</Field>
<button type="submit" disabled={submitting || pristine}>
Submit
</button>
</form>
)}
/>
</>
);
};
export default ReactFinalForm;
React Hook Form
Performant, flexible and extensible forms with easy-to-use validation.
- Official Web – https://react-hook-form.com/
- GitHub – https://github.com/react-hook-form/react-hook-form
- NPM – https://www.npmjs.com/package/react-hook-form
React Hook Form
is a lightweight library, and it leverages the power of React Hook for managing form state, validation, and submission. It offers a rich set of features.
- It supports external state management libraries by handling form states using hooks.
- It has a powerful internal validation and supports various third-party libraries like
Yup
. - It provides an API based on React Hooks. So it’s easy to learn.
- It supports both
controlled
anduncontrolled
form components.
React Hook Forms
is a fast-growing, well-popular, lightweight library in 2024. I know it is not as mature as the other two. But I can confirm when today (12/03/2024) React Hook Form
Library is the most popular library among these three.
And I can see most of the issue lists are solved on their GitHub page. ( Today (12/03/2024) only two issues are in open status. Other issues are closed – https://github.com/react-hook-form/react-hook-form/issues). So I can confirm the React Hook Form
community is active.
However I found a consideration,
React Hook Form
doesn’t support React class components.
Code sample
// react-hook-form.js
import React from 'react';
import { useForm } from 'react-hook-form';
const ReactHookForm = () => {
const {
register,
handleSubmit,
watch,
formState: { errors },
} = useForm();
// handle submit
const onSubmit = (data) => console.log(data);
console.log(watch('example')); // watch input value by passing the name of it
return (
<>
<h3>React Hook Form</h3>
<form onSubmit={handleSubmit(onSubmit)}>
<label htmlFor="firstName">First Name</label>
<input defaultValue="" {...register('firstName', { required: true })} />
{errors.firstName && <span>First Name is required</span>}
<label htmlFor="lastName">Last Name</label>
<input {...register('lastName', { required: true })} />
{errors.lastName && <span>Last Name is required</span>}
<label htmlFor="age">Age</label>
<input {...register('age', { required: true, pattern: /^[0-9]+$/i })} />
<>
{errors.age?.type === 'required' && 'Age is required'}
{errors.age?.type === 'pattern' && 'Age should be a number'}
</>
<label htmlFor="email">Email</label>
<input
{...register('email', {
required: true,
pattern: /^[A-Z0-9._%+-]+@[A-Z0-9.-]+\.[A-Z]{2,}$/i,
})}
/>
<>
{errors.email?.type === 'required' && 'Email is required'}
{errors.email?.type === 'pattern' && 'Invalid email address'}
</>
<input type="submit" />
</form>
</>
);
};
export default ReactHookForm;
Finally, My consideration is that React Hook Form
is a lightweight, performance-based library. It is ideal for both experienced and new developers who use react functional components only.
Bellow screenshot refers to the download trends for the past 5 years of Formik
, React Final Form
and, React Hook Form
.
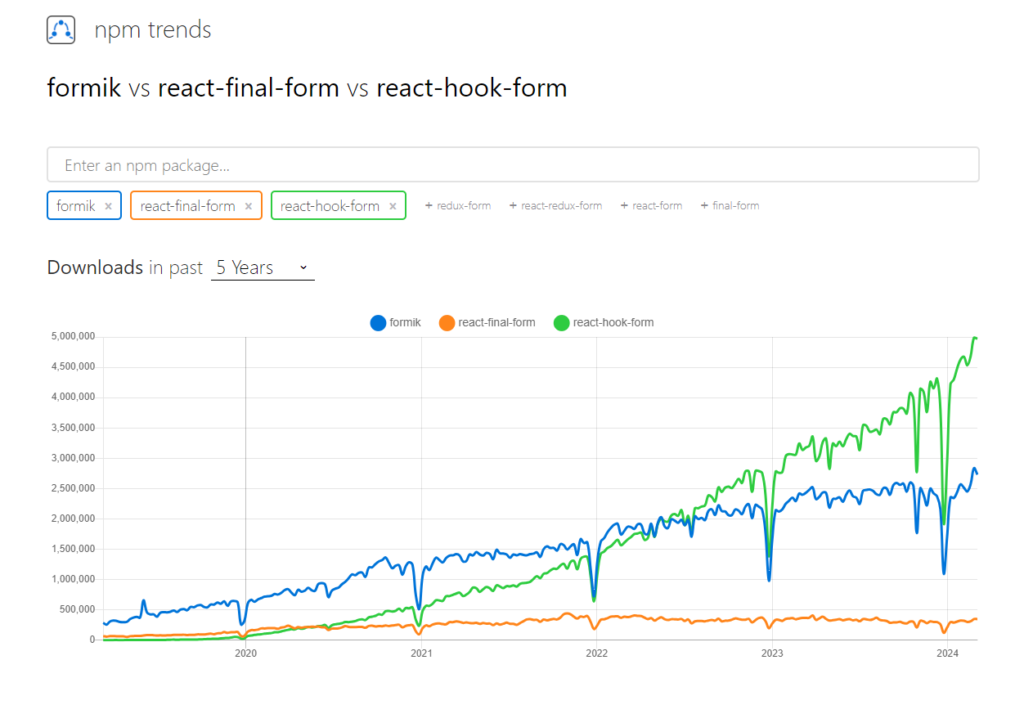
So according to the trends, we can say until mid-2022, Formik
was the most popular library. After mid-2022 the trend has changed. Now React Hook Form
is the most popular library.
Final comparison,
Feature | Formik | React Final Form | React Hook Form |
---|---|---|---|
Performance | Good | Good | Best |
Learning Curve | Moderate | Steeper | Easy |
UI library supports | Flexible | Flexible | Flexible |
Components type | Class and Functional | Class and Functional | Functional only |
Bundle size | Average bundle | Small bundle | Smallest bundle |
Conclusion
I did this comparison using my past few years of experience and with my own research. However, selecting the best library really depends on your requirements and preferences.
- React Hook Form offers a lightweight and performant solution, ideal for most form implementations.
- Formik offers a well-established codebase that supports both Class and Functional components.
- Then Finally React Final Form offers a better solid solution for complex scenarios.
Happy coding 🙂
Useful Links
Code Sample: https://github.com/chanakaHetti/react-form-research
Medium Article: https://medium.com/@chanakaH/choosing-the-right-react-form-library-for-2024-a7cb10931973